Tokenization and 3D Secure Support (Version 2)
Overview
Radial's foundational JavaScript library can be used to quickly integrate with the Radial Payments Framework in order to take advantage of Tokenization and 3D Secure (3DS) Support. This can be done by loading Radial's radial_payments.js and invoking the appropriate methods as needed.
Note: A similar process can be used to encrypt payment card numbers entered in mobile apps. For more information, see Encryption Support for Mobile Apps.
The radial_payments.js can be used for a wide variety of scenarios:
- Tokenization Only: This call is typically invoked when credit card information is captured without making a purchase. The webstore must use radial_payments.js and call appropriate functions to get token.
- Tokenization and 3DS Authentication: This call is typically invoked when the customer enters a credit card on the payment screen and places an order. In this case, the webstore is expected to call `tokenizeAndAuthorize` js method provided by Radial.
- 3DS Authentication with Saved Token: This call is typically invoked when the customer uses a saved card to place an order. In this case, the webstore is expected to invoke the `authorizeSavedToken` method provided by Radial passing in the account_token as an input parameter.
One of the following responses will be returned by Radial at the completion of the Authentication call: SUCCESS, FAILURE, ERROR, NOACTION.
If the response is SUCCESS or NOACTION (not enrolled), the account_token, which is the tokenized account number, is generally returned in the json data object to the callback function provided by the webstore when invoking the appropriate methods.
Including radial_payments.js
When using radial_payments.js, you begin by including the library. Add this script tag to your page:
- Test Environment
<script src=http://tst.payments.radial.com/hosted-payments/v2.0/radial_payments.js></script>
- Production Environment
<script src=https://hostedpayments.radial.com/hosted-payments/v2.0/radial_payments.js></script>
Webstore needs to add a div tag with the id="authentication-container", where they want to show the authentication popup on the checkout/payment
<div id="authentication-container"></div>
Tokenization and 3DS Authentication

Step #1: Nonce Generation
Nonce generation is a server-to-server call. For security purpose, Radial must authenticate the webstore prior to exchange any data. To facilitate authentication, Radial exposes a HTTPS REST endpoint where a webstore can submit its credentials in the request body.
During Radial Integration, each webstore will be provided with authentication credentials. The webstore must submit these credentials in the body of the nonce generation request.
Use these environment-specific URLs to generate a nonce:
- Test Environment
https://tst.payments.radial.com/hosted-payments/auth/nonce
- Production Environment
https://hostedpayments.radial.com/hosted-payments/auth/nonce
The following is an example of a nonce generation request body.
{
"username" : "username",
"password" : "password",
}
The nonce generation call provides a response similar to the following.
{
"nonce": "eyJhbGciOiJIUzUxMiJ9.eyJzdWIiOiJUUlVTMiIsImF1ZCI6IndlYiIsImV4cCI6MTU4MjE5NzcxOCwiaWF0IjoxNTgyMTk3NDE4fQ.aaXjnv9SPcKKoOt4k1jzM_tTisgNSZ6OoaNy43SRTI9LPo9APHV-BT1zej7vSCl694qzhJDuqvWyoqYC9ggOow" ,
"expires_in_seconds": 300
}
Note: The nonce obtained has an expiration period associated with it. Currently, the expiration duration is set as 300 seconds. But this expiration period can vary from time to time based on security requirements. If the nonce times out, you will receive an InvalidLoginError(50002) as a response for subsequent calls. If that occurs, the webstore can make a new server to server authentication call to get a new nonce.
The webstore must capture the response and send the information to the Radial. The nonce received in the response is required invoke/use the JavaScript library. The webstore must ensure the nonce value is available in the response.
In case of error, the payload below is returned:
{
"error_code" : "50002",
"error_message" : "InvalidLoginError",
}
Below is a list of possible errors that can be returned.
Error Code | Error Message | Description |
---|---|---|
50002 | InvalidLoginError | The nonce used to connect to Radial is invalid or timed out. Renew the nonce and try again. |
50003 | RadialInternalError | There was an internal error in Radial's system. |
The following code snippet generates the nonce.
public class RestCallClient {
public static void main(String[] args) {
try {
URL url = new URL("https://tst.payments.radial.com/hosted-payments/auth/nonce");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
String input = "{\n" +
"\t\"username\": \"username\",\n" +
"\t\"password\" : \"password\",\n" +
"}";
OutputStream os = conn.getOutputStream();
os.write(input.getBytes());
os.flush();
System.out.println(conn.getResponseCode());
BufferedReader br = new BufferedReader(new InputStreamReader(
(conn.getInputStream())));
StringBuilder builder = new StringBuilder();
String output;
System.out.println("Output from Server .... \n");
while ((output = br.readLine()) != null) {
System.out.println(output);
builder.append(output);
}
System.out.println(builder);
JSONObject jsonObject = new JSONObject(builder.toString());
System.out.println(jsonObject.getString("nonce"));
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Step #2: Download radial_payments.js and Initialize JavaScript
Note: The JavaScript integration makes a cross-site call from the browser to Radial's server instead of the originating webstore server. This is a CORS request. The webstore should give Radial the domain names of servers for Javascript integration, so that Radial can whitelist the servers to enable the cross-site call. The domain name should be passed in the format of https://website.com
Radial hosts a JavaScript file, radial_payments.js, which provides the tokenization functionality. The nonce received in Step #1 is necessary for subsequent JavaScript calls from the browser. The webstore should download the radial_payments.js file using the script tag when the customer navigates to the checkout page. After downloading the radial_payments.js file, the webstore should invoke the Radial.setup() method by passing the nonce as illustrated in the following example. In other words, Radial.setup() should be invoked in the checkout page onload event.
<head>
<script src="http://tst.payments.radial.com/hosted-payments/v2.0/radial_payments.js"></script>
<script language="JavaScript">
function loadNonce() {
Radial.setup('<%=request.getAttribute("nonce")%>');
}
</script>
</head>
<body onload="loadNonce()">
Tokenization Only
After capturing card data, the webstore code should immediately call Radial's javascript method Radial.tokenize(), passing the card data.
onclick="Radial.tokenize('accountNumber', tokenizeCallBackHandler);"
The webstore must implement a tokenizationCallbackHandler() JavaScript method that will be called when the Radial.tokenize() receives a response. The webstore can handle the response as in the following example.
<script language=" javascript">
tokenizationCallbackHandler(data) {
switch(data.ActionCode){
case "SUCCESS":
alert(data.account_token);
break;
case "FAILURE":
alert(data.failure_code);
alert(data.failure_message);
break;
case "ERROR":
alert(data.error_code);
alert(data.error_message);
break;
}
</script>
In case of success, the webstore can collect the account_token from the json object.
There are several possible failure responses for this call. Failure can occur with an invalid credit card number or a card number that failed a luhn check. The webstore can prompt the user to correct the error and retry the request.
Below is a list of possible failures that can be returned:
Error Code | Error Message | Description |
---|---|---|
40001 | NonNumericDataException | The account number passed in was not numeric |
40002 | PanTooShortException | The account number passed in was too short. |
40003 | PanDoesNotPassLuhnCheckException | The account number passed in did not pass luhn check. |
40004 | PanTooLongException | The account number passed in was too long. |
40006 | TokenNotFoundException | When the token passed in does not exist in Radial System. |
Some errors can be caused by an issue on the network or with one of Radial's internal services. If one of these errors is returned, the webstore can implicitly retry the request without user intervention. The following errors fall into this category.
Error Code | Error Message | Description |
---|---|---|
50001 | TimeoutError | There was a time out attempting to contact Radial's payment service |
50002 | NonceTimedoutError | The nonce used to connect to Radial is invalid or timed out. Renew the nonce and try again. |
50003 | RadialInternalError | There was an internal error in Radial's system. |
It is suggested that the client can bypass 3DS and proceed with order checkout in all 5000* error cases except during InvalidLoginError and NonceTimeoutError (50002) scenarios.
Integration Flow
The sequence diagram below shows the series of system interactions in 3DS Tokenization.
Redirects v2.0Steps for both 3D Secure 1.0 and 3D Secure 2.0
- The browser requests the payment page from the webstore server.
- The webstore server sends a request to Radial with the username and password provided during Radial Integration.
- The webstore server receives nonce in Radial's response.
- The webstore server provides the payment page along with nonce, which are used to initialize the JavaScript (with the Radial.setup() method). Webstore server should invoke the Radial.setup() javascript method during the checkout page onload().
- The user enters the card details, and the browser sends a 3DS request to Radial(If tokenization is needed: Radial.tokenizeAndAuthorize(), if token was previously saved: Radial.authorizeSavedToken()).
- Radial makes a call to the 3D Secure Verification Provider.
- Radial receives a response from 3D Secure Verification Provider.
When response is for a redirect
Customer will be redirected to the issuer page, where authentication is done. The webstore needs to provide return url in request object, see the section below on the 3DSecure Json Object Format for furter details. Webstore needs to be able to handle the redirect on the server side for HTTPMethod.GET and HTTPMethod.POST (received for legacy 3DS1.0 integration) requests. Webstore can append query parameters it needs to the returnUrl (shopper ids, order ids, etc. Payments will support the query parameters, so the webstore can append what it needs in that url without worry.)
- Browser is redirected to proper url to complete authentication.
- Browser submits authentication and is redirected back to returnUrl that was provided in step 5 above.
Handling Redirect with Http GET
- Receive authentication result and paymentId that will be appended in the returnUrl.
- Submit the CreditCardAuthRequest to Radial using the paymentId and AuthenticationResult. The authenticationResult can be sent in the authenticationResult element as shown below
<AuthenticationResult>eHy7hysdakYTS67sASnnJJ778lasdujI9====</AuthenticationResult>
Handling Redirect with Http POST
- Receive authentication result as a payload with fields "MD" and "PaRes". These fields contains the encrypted authentication result and paymentId that will be appended in the returnUrl.
- Submit the CreditCardAuthRequest to Radial using the paymentId and authentication result. The MD and PaRes fields should be sent in the authenticationResult element as shown below.
<AuthenticationResult>LEGACY_REDIRECT&MD=euhGYTHSaSE1Sdaaf342&PaRes=sfgsHVDMWEU13nHVS24JUUY===</AuthenticationResult>
When response is for version 2.0
Customer will complete authentication in the pop-up. When authentication is done the authenitcationResult and paymentId will be sent back in the callback handler response. The Webstore must implement callback handler function and read PaymentId and AuthenticationResult to be passed in the CreditCardAuthRequest. The response will also contain the tokenized pan and orderId
- Browser displays a popup for authentication.
- Submit authentication to the card issuer.
- Receive result of authentication from card issuers.
- Submit CreditCardAuthRequest using PaymentId and AuthenticationResult (see section on Callback handler for how to obtain these).
Step #4: Accept Card Data and Invoke Authentication
As soon as card data is captured, the webstore client code should call Radial's javascript method 'Radial.tokenizeAndAuthorize()' or Radial.authorizeSavedToken() as described above, passing the card data and the order data as a jsonObject, as shown in the examples below:
Radial.tokenizeAndAuthorize('pan', jsonObject, tokenizeAndAuthorizeCallbackHandler);
Radial.authorizeSavedToken('pan', jsonObject, authorizeSavedTokenCallbackHandler);
The webstore must implement the CallbackHandler methods and invoke the authorization request with the results of the 3DS authentication.
The table below describes the fields in the json object to be passed in to the Radial.tokenizeAndAuthorize() or Radila.authorizeSavedToken js methods. Some fields are marked optional, but the values in these fields are important in the 3D Secure rule utilization. It is recommended that all of the listed fields are passed in, when possible.
Note regarding shipping address: In certain orders for electronic delivery items, shipping address may not be available. In such cases, it is recommended to send the billing address as the shipping address. In scenarios where the order is being shipped to multiple destinations, send the shipping address of the destination that receives the maximum order value.
3d Secure Json Object Format
Field Name | Description | Required | Field Length |
---|---|---|---|
amount.currencyCode | 3 character alpha code ISO-4217 currency code for the sale amount | Y | AN(3) |
amount.value | Unformatted total transaction amount without any decimals. Eg: $100.00 = 10000, $123.67 = 12367 | Y | N(20) |
ipAddress | The IP Address of the consumer. Note: IPv4 and IPv6 are supported | Y | AN(50) |
orderNumber | Order identifier. Please ensure that the same order number is used in all subsequent transactions for the same order, such as credit card auth request, risk assessment, and order create. If the order number in subsequent calls does not match this order number, it nullifies the effect of the 3DS fraud check. | Y | AN(50) |
billingInformation.person.name.firstName | Consumer's first name | Y | AN(50) |
billingInformation.person.name.middleName | Consumer's middle name | N | AN(50) |
billingInformation.person.name.lastName | Consumer's last name | Y | AN(50) |
billingInformation.person.gender | Consumer's gender | N | AN(1) |
billingInformation.person.dateOfBirth | Consumer's Birthdate. Should be sent in this format 01/01/2001 | N | AN(10) |
billingInformation.contactInformation.phoneNumber | Consumer's phone number for billing address. This should be unformatted without hyphens. Example: 222-234-5678 = 2222345678 | Y | AN(20) |
billingInformation.contactInformation.emailAddress | Consumer's email address | Y | AN(255) |
billingInformation.line1 | Consumer's billing address line 1 | Y | AN(50) |
billingInformation.line2 | Consumer's billing address line 2 | N | AN(50) |
billingInformation.city | Consumer's city of billing address | Y | AN(50) |
billingInformation.state | Consumer's billing state | N | AN(50) |
billingInformation.countryCode | Consumer's alpha 2 digit ISO 3166 country code. Example: United States = US | Y | AN(3) |
billingInformation.postalCode | Consumer's billing postal code | Y | AN(10) |
shippingInformation.person.name.firstName | Recepient's first name | Y | AN(50) |
shippingInformation.person.name.middleName | Recepient's middle name | N | AN(50) |
shippingInformation.person.name.lastName | Recepient's last name | N | AN(50) |
shippingInformation.person.gender | Recepient's gender | N | AN(1) |
shippingInformation.person.dateOfBirth | Recepient's Birthdate. Should be sent in this format 01/01/2001 | N | AN(10) |
shippingInformation.contactInformation.phoneNumber | Recepient’s phone number for shipping address. This should be unformatted without hyphens. Example: 222-234-5678 = 2222345678 | Y | AN(20) |
shippingInformation.contactInformation.emailAddress | Recepient’s Consumer's email address | Y | AN(20) |
shippingInformation.line1 | Recepient's address line1 | Y | AN(50) |
shippingInformation.line2 | Recepient's address line2 | N | AN(50) |
shippingInformation.city | Recepient's address city | Y | AN(50) |
shippingInformation.state | Recepient's address state | N | AN(50) |
shippingInformation.countryCode | Recepient's alpha 2 digit ISO 3166 country code. Example: United States = US | Y | AN(3) |
shippingInformation.postalCode | Recepient's postal code | Y | AN(10) |
paymentMethod.tenderType | 2 digit the card type used, Visa (VC) Amex (AM) Discover(DC), Mastercard (MC), Klarna (KL), Alipay (AL) | N | AN(2) |
paymentMethod.cardDetails.rawCardNumber | This is required for credit card payments. card number being authenticated/authorized. Required to be sent when call tokenizeAndAuthorize method. | N | AN(4) |
paymentMethod.cardDetails.tokenizedCardNumber | This is required for credit card payments. tokenized card number being authenticated/authorized.Required to be sent when call authorizeSavedToken method. | N | AN(4) |
paymentMethod.cardDetails.expiryMonth | This is required for credit card payments.The expiry month of the card being authenticated/authorized. Formatted MM Eg. January = 01 | N | AN(2) |
paymentMethod.cardDetails.expiryYear | This is required for credit card payments.The expiry year of the card being authenticated/authorized. Formatted YYYY Example YYYY= 2016 | N | AN(4) |
paymentMethod.cardDetails.securityCode | This is required for credit card payments.The CVV of the card being authenticated/authorized | N | AN(4) |
recurringDetails.recurringEnabled | true if recurring payment is enabled for this order | N | AN(5) |
recurringDetails.frequency | The frequency of the recurring payment.Allowed values ANNUALLY, HALF_YEARLY, QUARTERLY, MONTHLY, BI_WEEKLY, WEEKLY, DAILY | N | AN(25) |
recurringDetails.recurrenceType | The type of recurrrence. INITIAL, SUBSEQUENT, ONCE | N | AN(25) |
recurringDetails.recurrenceId | The identifier of this recurrence payment. Webstore can send unique values for this field | N | AN(500) |
recurringDetails.paymentDueDate | The due date of the recurring payment.Should be in the format 12-MAR-2022 | N | AN(15) |
recurringDetails.startDate | The startDate of the recurrence.Should be in the format 12-MAR-2022 | N | AN(15) |
recurringDetails.endDate | The endDate of the recurrence. Should be in the format 12-MAR-2022 | N | AN(15) |
lineItems.type | The type of the line items | N | AN(15) |
lineItems.reference | The reference of the line items | N | AN(50) |
lineItems.name | The name of the line items | N | AN(250) |
lineItems.imageUrl | The url of the image | N | AN(250) |
lineItems.productUrl | The url of the product | N | AN(15) |
lineItems.quantity | The number of the products | N | AN(15) |
lineItems.unitPrice | The amount of the product | N | AN(15) |
lineItems.totalAmount | The sum of amount of all quantities * unitprices | N | AN(500) |
lineItems.taxAmount | The taxAmount of the product | N | AN(500) |
lineItems.totalTaxAmount | The total tax amount. | N | AN(500) |
lineItems.totalDiscountAmount | The discount amount of the product if any | N | AN(500) |
returnUrl | The return URL in (https://www.radial-demo-store.com/checkout?shopperId=123&orderId=345)to which the customer will be redirected after completing the authentication in a 3rd party website. This URL have requestParameter appended into. Required for 3DS1.0 version | Y | AN(500) |
originUrl | The domain of the URL that will be white listed. This URL is required for 3DS authentication integrations. Otherwise the authentication components may not work. | Y | AN(500) |
merchantCode | The radial identifier of the merchant code. | N | AN(500) |
Sample jsonObject Request with all elements
{
"amount": {
"value": 12345,
"currencyCode": "USD"
},
"taxAmount": {
"value": 1000,
"currencyCode": "USD"
},
"ipAddress": "IpAddress",
"orderNumber": "OrderNumber",
"browserInformation": {
"screenWidth": 20,
"screenHeight": 40,
"colorDepth": 40,
"userAgent": "userAgentTestData",
"acceptHeader": "headerData",
"timeZoneOffset": 0,
"language": "languageTestData",
"javaEnabled": true
},
"billingInformation": {
"person": {
"name": {
"firstName": "BillingFirstName",
"middleName": "BillingMiddleName",
"lastName": "BillingLastName"
},
"gender": "M",
"dateOfBirth": "01/01/2001"
},
"contactInformation": {
"phoneNumber": "BillingPhone",
"emailAddress": "Email"
},
"line1": "Address1",
"line2": "Address2",
"city": "BillingCity",
"state": "BillingState",
"postalCode": "BillingPostalCode",
"countryCode": "BillingCountryCode"
},
"shippingInformation": {
"person": {
"name": {
"firstName": "ShippingFirstName",
"middleName": "ShippingMiddleName",
"lastName": "ShippingLastName"
},
"gender": "M",
"dateOfBirth": "01/01/2001"
},
"contactInformation": {
"phoneNumber": "ShippingPhone",
"emailAddress": "Email"
},
"line1": "ShippingAddress1",
"line2": "ShippingAddress2",
"city": "ShippingCity",
"state": "ShippingState",
"postalCode": "ShippingPostalCode",
"countryCode": "ShippingCountryCode"
},
"paymentMethod": {
"tenderType": "TenderType",
"cardDetails": {
"rawCardNumber": "RawPan",
"tokenizedCardNumber": "TOKEN",
"expiryMonth": "CardExpMonth",
"expiryYear": "CardExpYear",
"securityCode": "1234"
}
},
"recurringDetails": {
"recurringEnabled": true,
"frequency": "ANNUALLY",
"recurrenceType": "INITIAL",
"recurrenceId": "98765",
"paymentDueDate": "12-MAR-2022",
"startDate": "12-FEB-2022",
"endDate": "12-MAR-2022"
},
"lineItems": [
{
"type": "physical",
"reference": "coolthing1",
"name": "cool thing 1",
"imageUrl": "http://www.estore.com/image1.png",
"productUrl": "http://lwww.estore.com/product1/",
"quantity": 1,
"unitPrice": 50000,
"totalAmount": 50000,
"taxRate": 200,
"totalTaxAmount": 1000,
"totalDiscountAmount": 8000
}
],
"merchantCode": "MerchantCode",
"originUrl" : "localhost:8080",
"returnUrl": "www.mystore.com/checkout?shopperId=123&orderId=abcdef"
}
Radial handles the handshake with the 3DS provider and redirects the browser to an 3DS authentication window that looks like the example below:
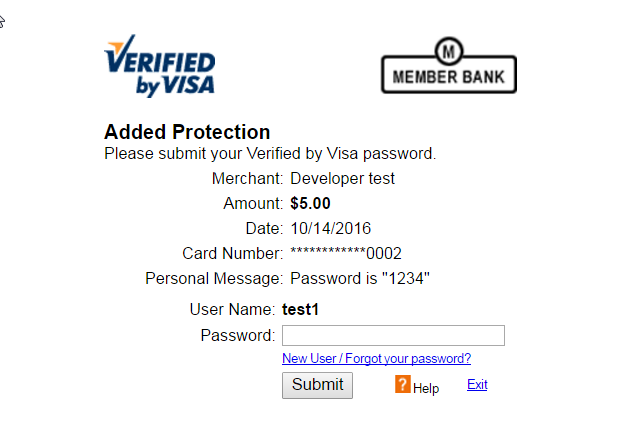
The user is prompted for authentication information.
Upon completion of the process, Radial calls the callbackFunction provided during the authentication call. The webstore must implement a tokenizeAndAuthorizeCallbackHandler() JavaScript function. 'Radial.tokenizeAndAuthorize()/Radial.authorizeSavedToken()' call sends a response with the authentication result and other details as mentioned below in the Response Format section. The webstore can handle the response as shown below:
<script language="javascript">
tokenizeAndAuthorizeCallbackHandler (data) {
switch(data.ActionCode){
// Handle successful authentication scenario
case "SUCCESS":
alert(data.account_token);
// Use these values when creating the CreditCardAuthRequest
console.log(data.paymentReferenceId);
console.log(data.authenticationResult);
break;
// Handle unenrolled scenario (treat same as success)
case "NOACTION":
alert(data.account_token);
break;
// Handle authentication failed or error encounter scenario
case "FAILURE":
alert(data.failure_code);
alert(data.failure_message);
break;
// Handle service level error
case "ERROR":
alert(data.error_code);
alert(data.error_message);
break;
}
</script>
In case of SUCCESS or NOACTION, the webstore can collect the account_token from the json object and proceed. SUCCESS and NOACTION codes indicate everything is good with respect to the card, and the webstore can proceed with the transaction.
Response Format
The response object returned in the callback handler is in json format and in general has the format shown below.
{
"account_token": "tokenizedPan",
"paymentReferenceId" : "paymentId",
"orderId" : "orderId",
"authenticationResult" : "placeholder",
"responseCode":"SUCCESS"
}
In case of failure, it could be because of an invalid credit card number or a credit card number that failed a luhn check. The webstore can prompt the user to correct the error and retry the request.
Below is a list of possible failures that can be returned:
Error Code | Error Message | Description | ||
---|---|---|---|---|
40001 | NonNumericDataException | The account number passed in was not numeric | ||
40002 | PanTooShortException | The account number passed in was too short. | ||
40003 | PanDoesNotPassLuhnCheckException | The account number passed in did not pass luhn check. | ||
40004 | PanTooLongException | The account number passed in was too long. | ||
40005 | Incomplete3dsData | The json object passed in does not have all the required fields. | ||
40006 | TokenNotFoundException | When the token passed in does not exist in Radial System. | ||
40007 | Authentication failure/Signature verification failure. | When user authentication in the 3DS challenge popup fails or there is a signature verification failure at 3D Secure Verification Provider end. | ||
40008 | BasicParamSetupException | Before calling this method, some basic parameters need to be set |
Some errors can be caused by an issue on the network or with one of Radial's internal services. If one of these errors is returned, the webstore can implicitly retry the request without user intervention. The following errors fall into this category.
Error Code | Error Message | Description |
---|---|---|
50001 | TimeoutError | There was a time out attempting to contact Radial's payment service |
50002 | InvalidLoginError | The nonce used to connect to Radial is invalid or timed out. Renew the nonce and try again. |
50003 | RadialInternalError | There was an internal error in Radial's system. |
50004 | InvalidJWTError | The JWT used to connect to Radial is invalid. Get a fresh jwt and try again. |
50005 | 3dsGatewayTimeoutError | The 3DS gateway timed out. At this point, the webstore can choose to take the order without 3DS authentication if it wishes or retry the authentication again. |
50006 | 3dsGatewayInternalError | There was an internal error with the 3DS gateway. |
Note: It is suggested that the client can bypass 3DS and proceed with order checkout in all 5000* error cases except during InvalidLoginError and NonceTimeoutError (50002) scenarios.
Testing JavaScript API Integration
Radial offers a set of mock card numbers that can be used when testing your integration with Radial's JavaScript APIs. For details, see Testing JavaScript API Integration.
3d Secure Json Object Format:
If the authorization is not giving the expected results (approve, decline, etc) please check with the Radial Payments to make sure proper responses have been mapped.
When prompted for 3D Secure 2 text challenges, use the following credentials: For native mobile integrations, use password: 1234. For web and mobile browser integrations, use user: user and password: password
Scenario | PAN | PIN | Expiration Date |
---|---|---|---|
Amex Redirect | 345177925488348 | 7373 | 03/2030 |
Visa Redirect | 4212345678901237 | 737 | 03/2030 |
Card Not Enrolled Redirect | 4199350000000002 | 737 | 03/2030 |
Amex 3DS v2 | 371449635398431 | 7373 | 03/2030 |
Discover 3DS v2 | 6011111111111117 | 737 | 03/2030 |
Visa 3DS v2 | 4917610000000000 | 737 | 03/2030 |
3DS v2 Not Enrolled | 4199350000000002 | 737 | 03/2030 |
3DS v2 Error | 5201282999005515 | 737 | 03/2030 |